There is something mesmerizing about the demo in "WebGL Scroll Animations". Between all the calculations it does to create the unrolling, it uses the Y coordinate of the plane as an angle instead of a position.
The idea of highjacking the position value for your own ends is interesting, although usually, that end is calculating the position again. I do this a lot when making scrollers. Position them in the X axis next to each other, and then use the X axis as my angle for a distortion.
For the break-apart effect on the main page of the site, I did something similar with color. I needed centroids for each piece, but Big Bad Blender wouldn't allow me to export custom attributes to GLTF. What did I do? Encode them into the color attribute and use that instead.
course
Mastering ThreeJS Instancing for Creative Developers
EARLY BIRD DISCOUNT 20%! Learn how to create amazing demos with instancing in this fun exercise-centered ThreeJS course.
Learn More!Line post-processing
This week I was really into using a Pomodoro Clock, and also heavily inspired by Apple's price clock. My version of this is not only for numbers but the whole scene in the style.
First, it renders the time into a regular 2D canvas. The "Thunder" font I use on the site made this very easy because it almost made a square-shaped result.
The second step, render my scene in black and white with a fog to make the farther sections of the cube disappear.
In a postprocessing step, I draw the lines and use black-and-white results to make each section bigger. The tricky part is each "line section" has to sample from the same place for it to increase in size correctly.
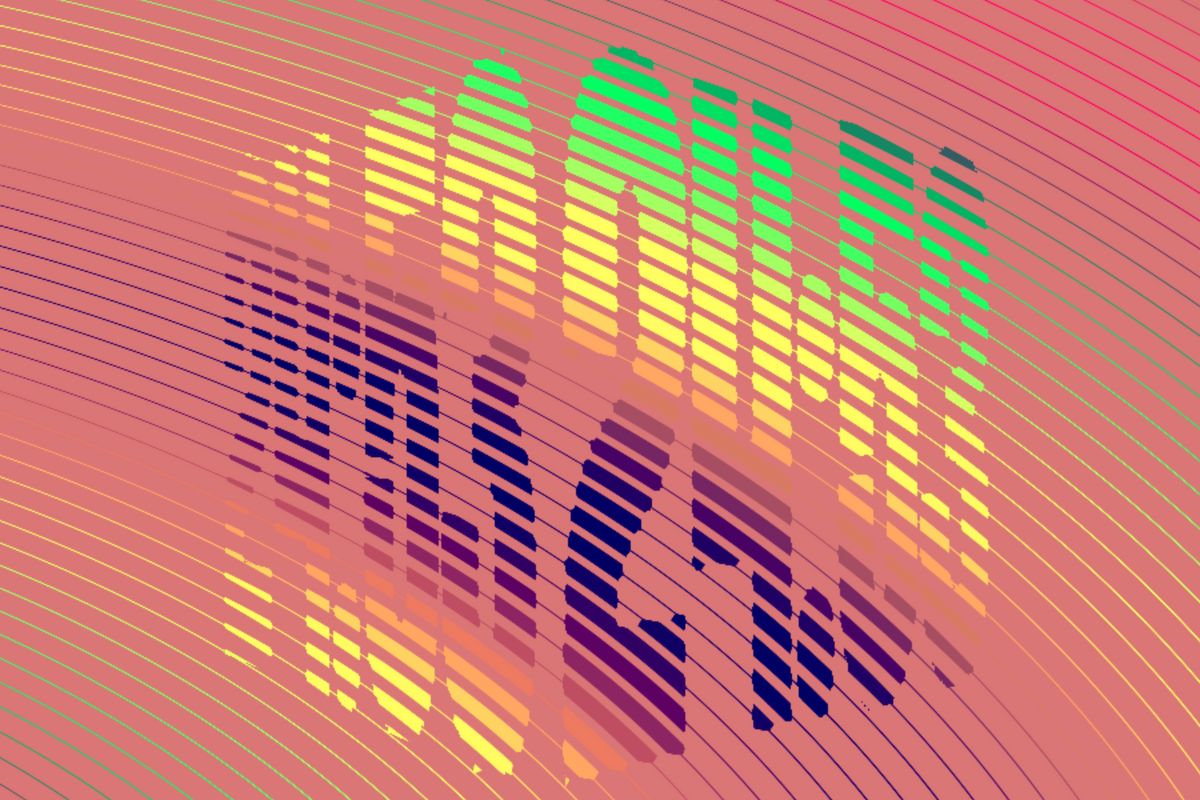
WebGL Scroll Animations - Unrolling
Lurking on the interwebs I found this How to roll up a Toilet paper unity question. The trick here is to take one coordinate, the Y coordinate, and use that as a startingRollAngle. Then, define a startingRollAngle to define what's the angle at the start of the Y coordinate.
With a progress uniform uRoll define how much rolling has been done. And use that uniform to calculate how big is the UnrolledAngle.
With these three values, you can get the arcLength between them, the arcLength to the end, and the arc length from the start to the current point. And use those to calculate the Z and Y coordinates again.
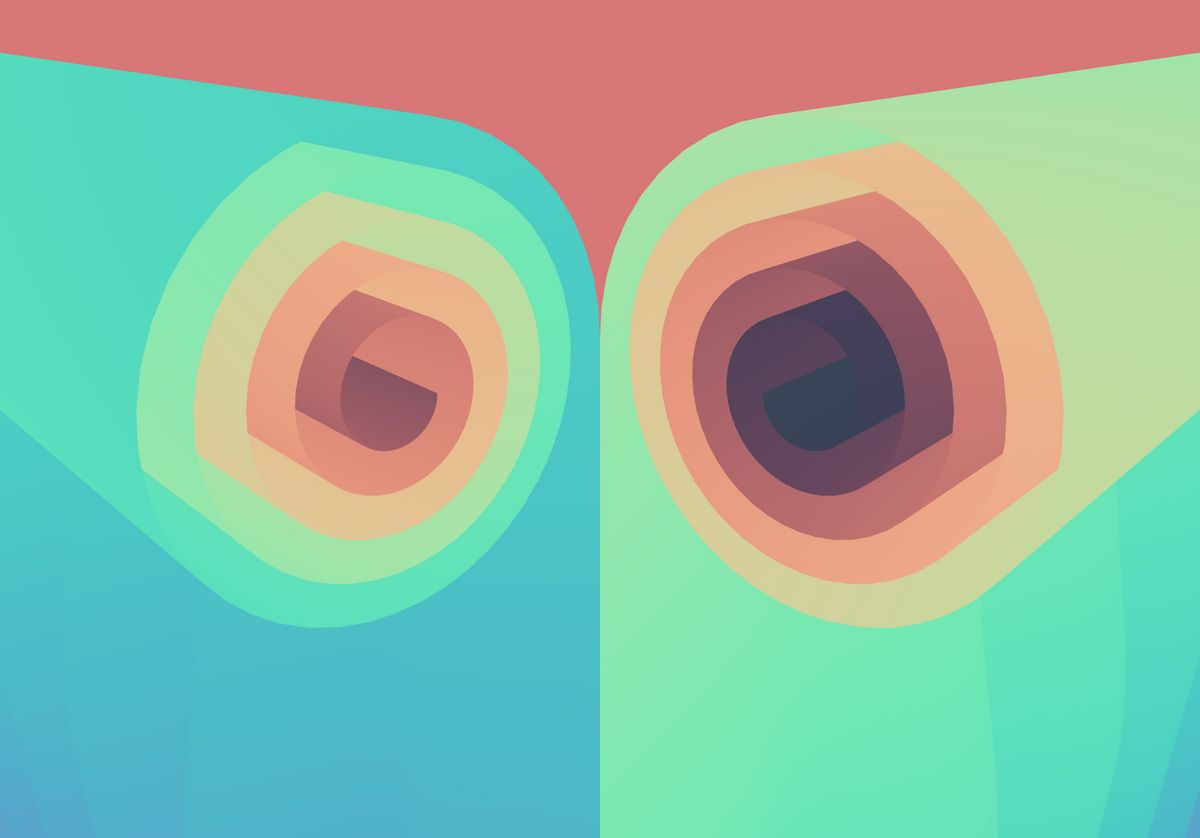
GLSL Dithering
No post-processing here. None. You can notice this yourself because the blob sometimes renders half pixels. These are two meshes: A fullscreen plane for the background, and a sphere with noise on the front. They apply dithering to themselves.
Both the plane and the blob calculate the screenUVs inside the fragment shader. Then, use those screenUVs for the 8x8 glsl-dithering function.
vec2 uv = (gl_FragCoord.xy / uResolution.xy) * (1./uAspect);
The half pixels look a bit odd, but saving a post-processing step is worth it.
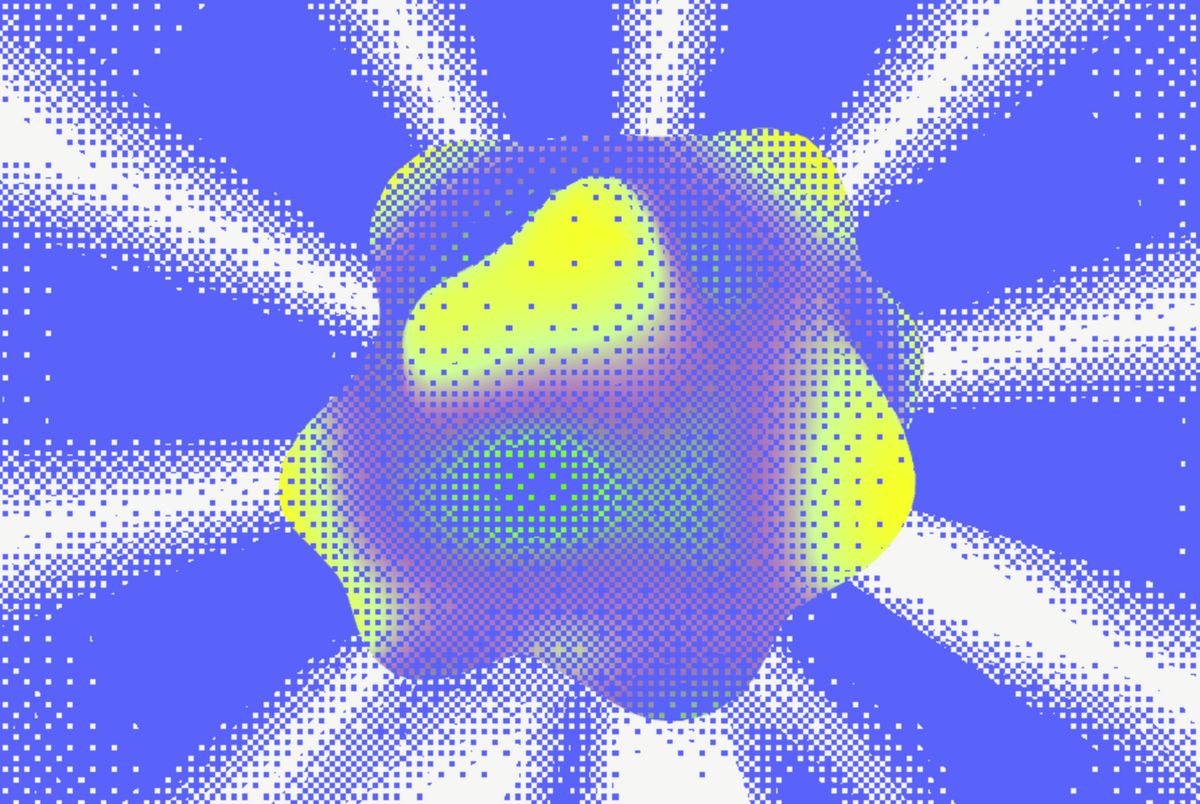
Fragment shader art
A single shader with a lot of over complications. This runs a loop inside the shader which adds more and more to a single variable. Then, use that variable to drive the color palette effect.
The intro/outro animation uses my all-time easiest and favorite trick: Calculate the grey color, and smoothstep over it.
float greyAnim = (anim.r + anim.g + anim.b)/3.;
float blendIntoBackground = smoothstep(0.,greyAnim, (1.-uVisibility))
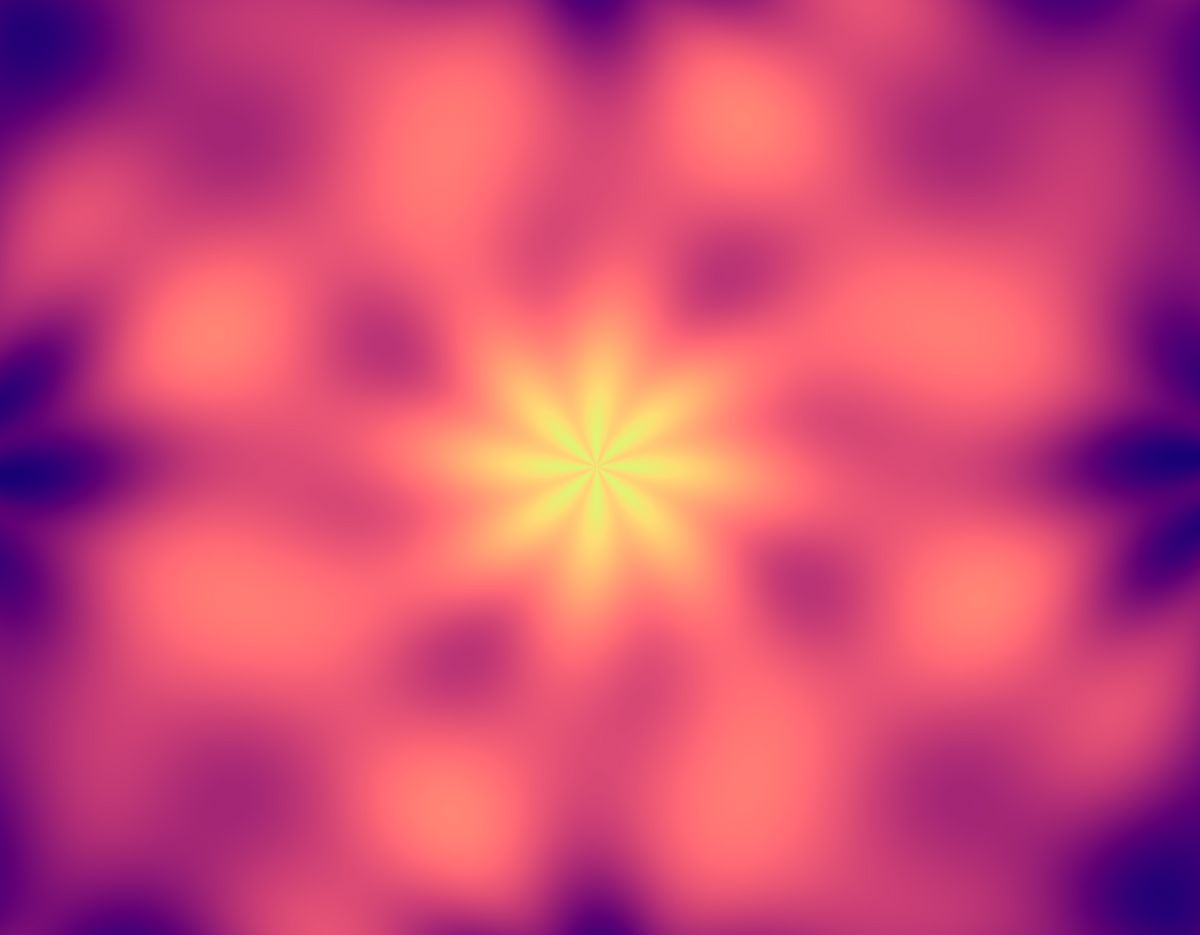