Usually Physics simulation occur in a different world to your regular scene. You have your render your scene, and then a physics world. The physics have a separate simplified world which is used to calculate the position of things and then transfer the position/updates to the render world, your scene objects.
This separation is crucial because you don't always want to run physics on the actual geometries you are working on because complex geometries require more computing. Usually, you run physics on simpler shapes that produce a good enough result.
sponsor
Become a sponsor with your sponsor spot here!
Promote your product/company in this spot to hundreds of creative developers interested in learning and creating. One spot per issue!
Learn More!Using CannonJS
Physics libraries take care of this world for you. The only thing you need is to create a world, and add bodies that match your scene objects. For simplicity, in my demo I attached each body to the corresponding mesh so it's easier to update them later on.
let world = new CANNON.World();
let sphere = new CANNON.Body({
mass: 1,
position: new CANNON.Vec3(0,0,0),
shape: new CANNON.Sphere(centerSize)
});
mesh.userData.body = sphere;
world.addBody(sphere)
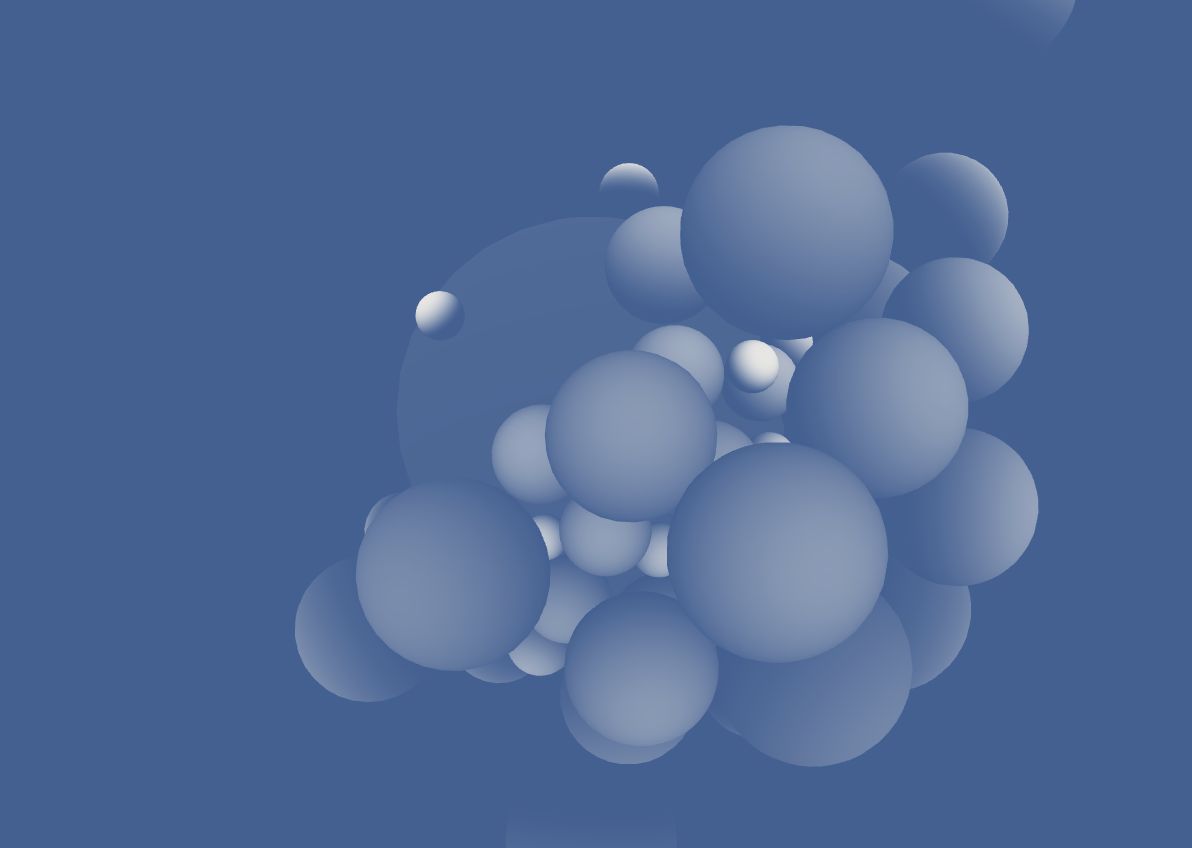
Constraints vs Forces
At first, I figured I'd made my demo with a bunch of constraints to the center sphere, I wanted all spheres to follow the center so a constrain made sense in my eyes. This didn't worked very well.
What did work is to calculate the direction towards the center_sphere, and apply a fraction of the distance as force instead of using constraints which I assume are for actually tied together things.
tempVec.copy(mesh.body.position)
tempVec.vsub(this.centerMesh.body.position, tempVec)
tempVec.mult(-4., tempVec)
mesh.body.applyForce(tempVec, new CANNON.Vec3(0,0,0))
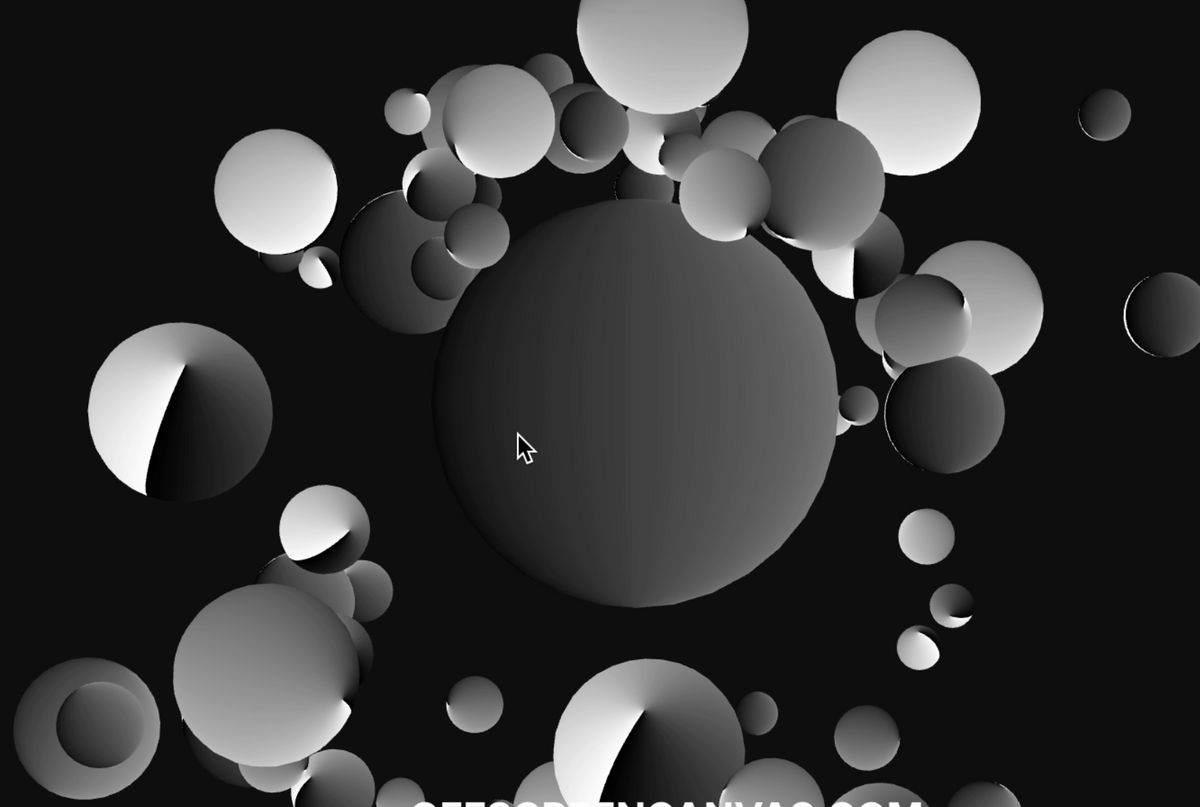
Click Explosion
Similar to following the center_sphere, I calculated the direction towards the center_sphere but then reversed it so it points away from the center_sphere. Then, I made the value even bigger to make them explode on click up.
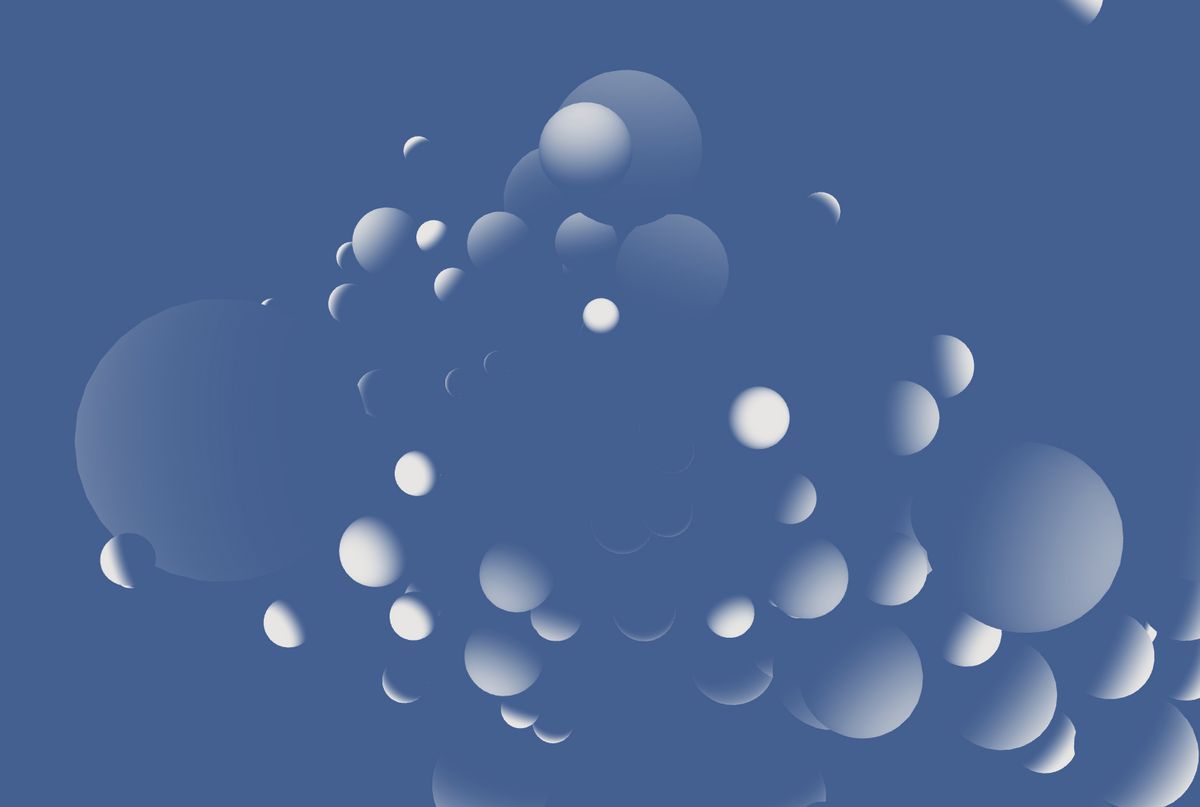
Tightly packed spheres
While adding forces made the spheres eventually reach the target, this would always take some time because of the velocity and the fact that it takes them time to slow down.
So, to quickly force them to stick together, when the mouse is down I manually modified the velocity of each body instead of adding force to reach the center. This meant that I didn't have that springiness of the force applied to the velocity. Instead, it's direct and as quick as I need it to be.
if(this.isDown){
let a = this.centerMesh.body.position.clone()
let pos = mesh.body.position.clone()
pos.mult(-1, pos)
a.vadd(pos, a)
a.mult(4, a)
mesh.body.velocity.copy(a)
}
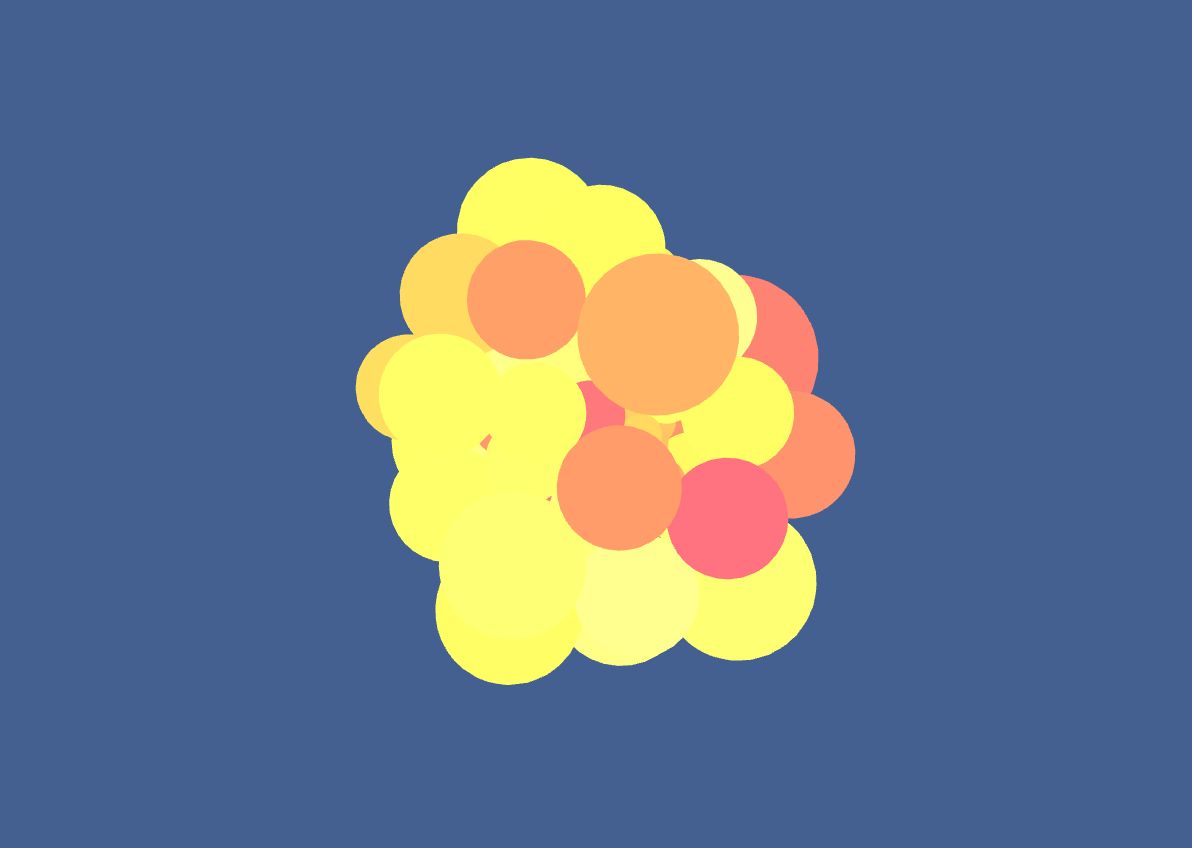